
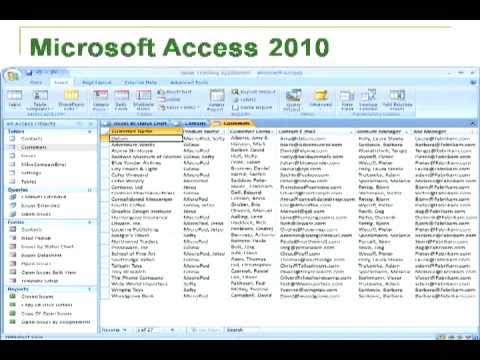
- #ACCESS DATABASE INSTALL#
- #ACCESS DATABASE DRIVERS#
- #ACCESS DATABASE DRIVER#
- #ACCESS DATABASE DOWNLOAD#
There’s no effective pandas method for this operation. To delete an entire table, it’s probably easier to just use the SQL way. cursor.execute('create table new_table_name (Ĭmit() Load Excel data into MS Access cursor.execute('select * into new_table_name' However, this is an empty table with no data. The below will create a new table with the name “new_table_name”, and specify the column names (column1, column2, column3, …) and their respective data types. When using SQL statements to modify the database, we have to commit the changes, otherwise, they will not be saved. The best part is that the result is in a pandas dataframe! import pandas as pdĭf = pd.read_sql('select * from table_name', conn) All we need is that SQL statement and the connection object, then pandas will extract everything (equivalent to fetchall()) for us. As you see below, we don’t have to worry about cursors or fetching results after execution. Similar to the method above, we also need a SQL statement, but it’s much easier. I personally prefer to use pandas to do the query, because most of the time we need to process the data after querying, so pandas is a natural choice. Rows = cursor.fetchall() Use pandas to query #Run SQL statementĬursor.execute('select * from table_name') If you need help with SQL the w3schools is a very good site that I used to learn SQL. This is not an SQL tutorial so we won’t cover the details.
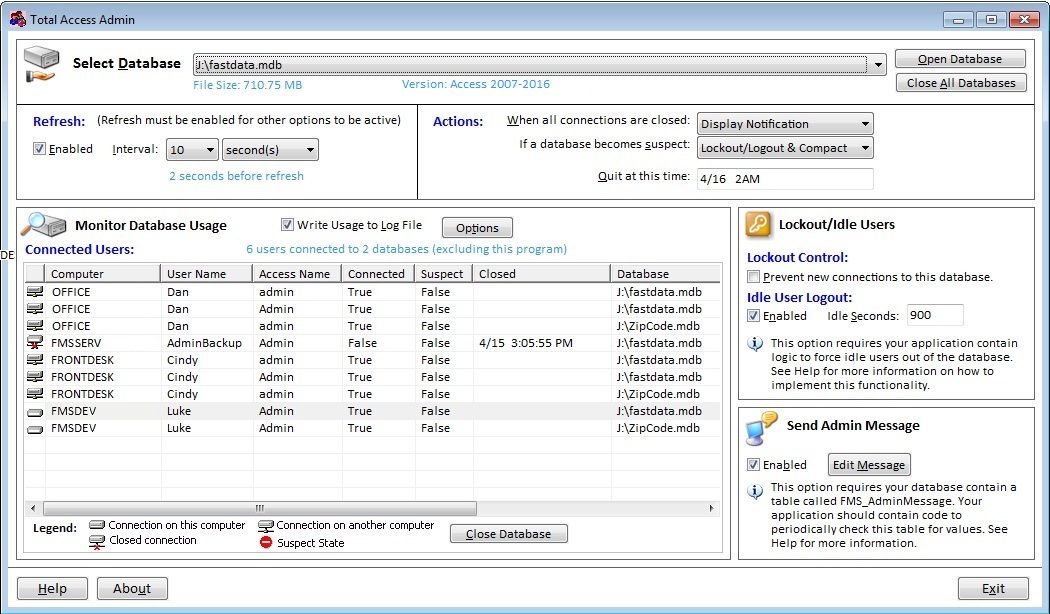
There’s no exception even if we are using Python to “talk” to the database. To interact with a database, we have to use a language that it understands. Note a “cursor” is an object used to execute SQL statements. But seriously, if we can use Python why even bother with Access queries ¯\_(ツ)_/¯ cursor = conn.cursor()įor i in cursor.tables(tableType='TABLE'):įor i in cursor.tables(tableType='VIEW'): We can loop through all the tables inside the Access database and filter by data tables (‘TABLE’) or queries (‘VIEW’). R'DBQ=E:\PythonInOffice\python_connect_to_ms_access\database.accdb ')Ĭonn = nnect(conn_str) Find all tables and queries in the MS Access database
#ACCESS DATABASE DRIVER#
For MS Access, we also need to specify the type of ODBC driver (32bit vs 64bit) in the connection string.Īlso make sure you close the MS Access database before making the connection, otherwise there will be an error. To connect to a database, we need a connection string, basically a text pointer that tells Python where to find the database. The above result shows that my computer has the new 64-bit Access (and driver).
#ACCESS DATABASE DRIVERS#
This list comprehension iterates through all available ODBC drivers and only returns the ones that start with “Microsoft Access Driver”. To check which version of the Access ODBC driver is on your computer, do the following in Python: >import pyodbc
#ACCESS DATABASE DOWNLOAD#
In case you don’t have the driver, you can download a standalone version on Microsoft’s website: Your machine should already have one of the drivers if you have MS Office installed. New Driver (64-bit) – Microsoft Access Driver ( *.mdb, *.accdb): works with 64-bit Python.Old Driver (32-bit) – Microsoft Access Driver ( *.mdb): works with 32-bit Python.The reason is that there are two different Access ODBC drivers from Microsoft: Mixing up a 64-bit Python with 32-bit Access will throw an error when you try to connect. One thing to note upfront, if you have 64-bit MS Access, you’ll want to use the 64-bit Python for this exercise. TL DR – You need 32-bit Python for 32-bit Access, or 64-bit Python for 64-bit Access.
#ACCESS DATABASE INSTALL#
Install pyodbc and check ODBC driver version pip install pyodbc
